如今,在涉及与不提供测试工具的各种云服务有关的代码测试方面,存在一个主要问题。例如,尽管您可能具有用于本地发布/订阅测试的工具,包括Docker映像,但是您可能没有可以模拟BigQuery的任何东西。
由于测试是要求的一部分,因此在CI作业方面会引起问题,但是在使用实际服务进行测试时可能会遇到障碍。 情况是,您确实需要涵盖所有需要涵盖的悲观情况(例如超时)。
这就是Hoverfly可以提供帮助的地方。
Hoverfly是一个轻量级的开源API模拟工具。 使用Hoverfly,您可以创建应用程序所依赖的API的逼真的模拟
我们的第一个示例将仅与模拟Web服务器有关。 第一步是添加Hoverfly依赖项。
< dependencies >
< dependency >
< groupId >io.specto</ groupId >
< artifactId >hoverfly-java</ artifactId >
< version >0.12.2</ version >
< scope >test</ scope >
</ dependency >
</ dependencies >
除了使用Hoverfly码头工人镜像外,我们还将使用Java库来获得更多的灵活性。
在配置Hoverfly模拟模式时,我们有两个选择。 一种是通过Java dsl,另一种是通过json。让我们同时介绍一下。
下面的示例使用Java DSL。 我们在8085上启动hoverfly并加载此配置。
class SimulationJavaDSLTests {
private Hoverfly hoverfly;
@BeforeEach
void setUp() {
var simulation = SimulationSource.dsl(service( " http://localhost:8085 " )
.get( "/user" )
.willReturn(success( "{\"username\":\"test-user\"}" , "application/json" )));
var localConfig = HoverflyConfig.localConfigs().disableTlsVerification().asWebServer().proxyPort( 8085 );
hoverfly = new Hoverfly(localConfig, SIMULATE);
hoverfly.start();
hoverfly.simulate(simulation);
}
@AfterEach
void tearDown() {
hoverfly.close();
}
@Test
void testHttpGet() {
var client = HttpClient.newHttpClient();
var request = HttpRequest.newBuilder()
.uri(URI.create( " http://localhost:8085/user " ))
.build();
var res = client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.join();
Assertions.assertEquals( "{\"username\":\"test-user\"}" ,res);
}
}
现在让我们对Json做同样的事情。 无需手动使用json进行操作,我们可以使代码为我们完成工作。
var simulation = SimulationSource.dsl(service( " http://localhost:8085 " )
.get( "/user" )
.willReturn(success( "{\"username\":\"test-user\"}" , "application/json" )));
var simulationStr = simulation.getSimulation()
System.out.println(simulationStr);
我们可以获得由Java DSL生成的JSON。 结果将是这样。
{
"data" : {
"pairs" : [
{
"request" : {
"path" : [
{
"matcher" : "exact" ,
"value" : "/user"
}
],
"method" : [
{
"matcher" : "exact" ,
"value" : "GET"
}
],
"destination" : [
{
"matcher" : "exact" ,
"value" : "localhost:8085"
}
],
"scheme" : [
{
"matcher" : "exact" ,
"value" : "http"
}
],
"query" : {},
"body" : [
{
"matcher" : "exact" ,
"value" : ""
}
],
"headers" : {},
"requiresState" : {}
},
"response" : {
"status" : 200,
"body" : "{\"username\":\"test-user\"}" ,
"encodedBody" : false ,
"templated" : true ,
"headers" : {
"Content-Type" : [
"application/json"
]
}
}
}
],
"globalActions" : {
"delays" : []
}
},
"meta" : {
"schemaVersion" : "v5"
}
}
让我们将其放置在测试的资源文件夹下,名称为Simulation.json。
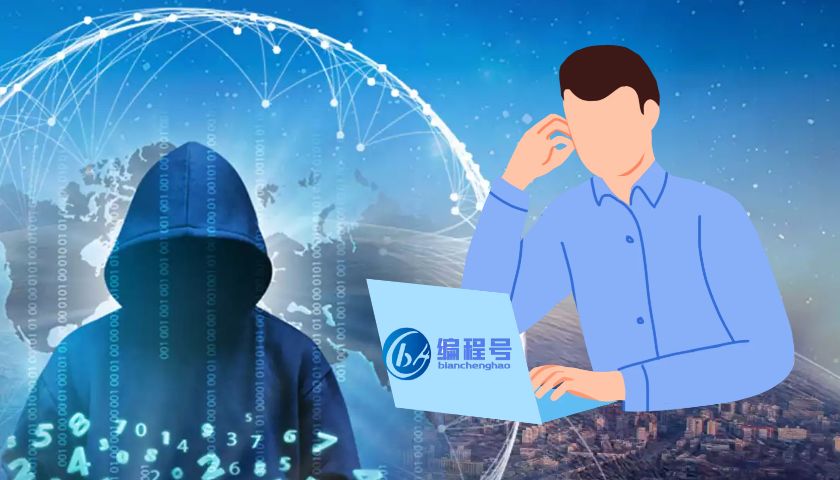

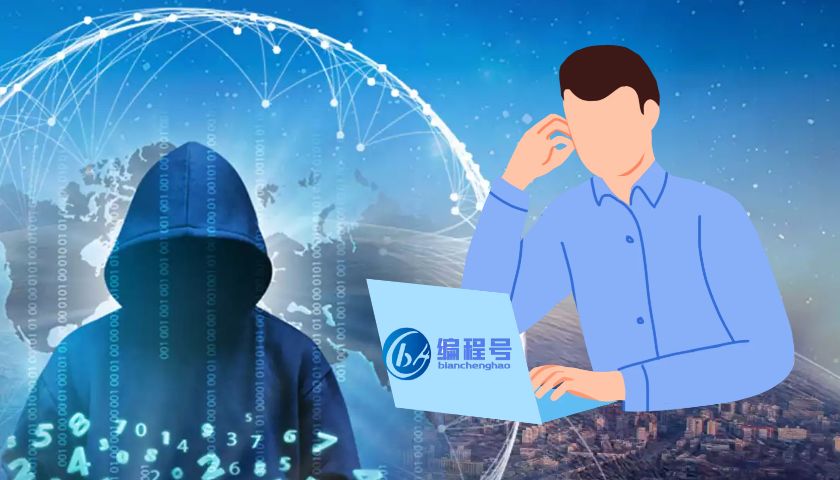
通过一些代码更改,我们可以获得完全相同的结果。
public class SimulationJsonTests {
private Hoverfly hoverfly;
@BeforeEach
void setUp() {
var simulationUrl = SimulationJsonTests. class .getClassLoader().getResource( "simulation.json" );
var simulation = SimulationSource.url(simulationUrl);
var localConfig = HoverflyConfig.localConfigs().disableTlsVerification().asWebServer().proxyPort( 8085 );
hoverfly = new Hoverfly(localConfig, SIMULATE);
hoverfly.start();
hoverfly.simulate(simulation);
}
@AfterEach
void tearDown() {
hoverfly.close();
}
@Test
void testHttpGet() {
var client = HttpClient.newHttpClient();
var request = HttpRequest.newBuilder()
.uri(URI.create( " http://localhost:8085/user " ))
.build();
var res = client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.join();
Assertions.assertEquals( "{\"username\":\"test-user\"}" ,res);
}
}
有时也需要组合仿真,而不管它们是json还是Java。 也可以通过加载多个仿真来简化此过程。
@Test
void testMixedConfiguration() {
var simulationUrl = SimulationJsonTests. class .getClassLoader().getResource( "simulation.json" );
var jsonSimulation = SimulationSource.url(simulationUrl);
var javaSimulation = SimulationSource.dsl(service( " http://localhost:8085 " )
.get( "/admin" )
.willReturn(success( "{\"username\":\"test-admin\"}" , "application/json" )));
hoverfly.simulate(jsonSimulation, javaSimulation);
var client = HttpClient.newHttpClient();
var jsonConfigBasedRequest = HttpRequest.newBuilder()
.uri(URI.create( " http://localhost:8085/user " ))
.build();
var userResponse = client.sendAsync(jsonConfigBasedRequest, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.join();
Assertions.assertEquals( "{\"username\":\"test-user\"}" ,userResponse);
var javaConfigBasedRequest = HttpRequest.newBuilder()
.uri(URI.create( " http://localhost:8085/admin " ))
.build();
var adminResponse = client.sendAsync(javaConfigBasedRequest, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.join();
Assertions.assertEquals( "{\"username\":\"test-admin\"}" ,adminResponse);
}
就是这样,我们已经做好了继续探索Hoverfly及其功能的设置。
版权声明:本文内容由互联网用户自发贡献,该文观点仅代表作者本人。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如发现本站有涉嫌侵权/违法违规的内容, 请发送邮件至 举报,一经查实,本站将立刻删除。
如需转载请保留出处:https://bianchenghao.cn/34124.html